SharpLeaf Reference > Table Class Table Class
Build a table of text data for SharpLeaf.
public class Table
Example
A Table is generally created in three phases:
- Set up table-wide settings
- Flow content into cells, possibly changing settings on the way
- Include table into SharpLeaf report with the IncludeTable method.
// Set up table-wide settings
tb = new Table();
tb.SetTitles(1,1); // 1 column and 1 row of titles
tb.SetOffset(1,0); // Offset table box to exclude 1 column and 0 row
tb.SetCellMargins(10); // More space around cell content
tb.Box = new BoxStyle(3,Color.Navy,LineStyle.Solid,10,Color.Navy,FillStyle.Saturate10,true);
tb.SetRowGrid(0); // Disable row grid
// Go through cells, possibly changing settings on the way
tb.SetCellFont(new FontType("Times",24,FontStyle.Bold));
tb.AddRows(new string[]{"","Column 1","Column 2","Column 3"});
tb.AddColumns(new string[]{"Row 1","Row 2","Row 3","Row 4"});
tb.SetCellFont(new FontType("Arial",20));
tb.AddRows(new int[][]{new int[]{101,201,301},new int[]{102,202,302},new int[]{103,
203,303},new int[]{104,204,304}});
// Include table in SharpLeaf flow
lf.IncludeTable(tb);
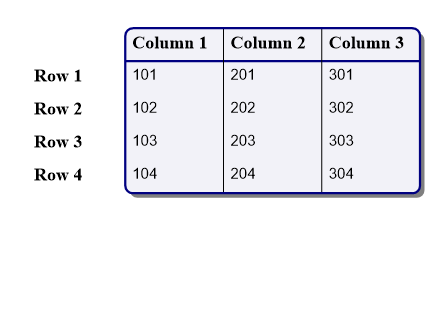
Have a look at the Table tutorials for more examples.
Constructors
Table Instance PropertiesBox | BoxStyle for whole table (null for no box) (default is 1-pt black unfilled) | Caption | Text displayed at the top of table on every sub-page | CaptionAlignment | Horizontal Alignment of Caption (Left by default) | CaptionFont | FontType for Table Caption (default is null to use working ParagraphStyle) | Column | Column index of the current cell pointer | ColumnOverwrite | Overwrite column settings when re-visiting columns in future cells (default is false) | Columns | Number of columns in current table (ReadOnly) | Footnote | Text displayed once at the end of the Table | FootnoteAlignment | Horizontal Alignment of Footnote (Left by default) | FootnoteFont | FontType for Table Footnote (default is null to use working ParagraphStyle) | Heading | Text displayed once at the beginning the Table | HeadingAlignment | Horizontal Alignment of Heading (Left by default) | HeadingFont | FontType for Table Heading (default is null to use working ParagraphStyle) | MergeOrder | Set cell merging order when both columns and rows can be merged (default is true for columns first) | Row | Row index of the current cell pointer | RowOverwrite | Overwrite row settings when re-visiting rows in future cells (default is false) | Rows | Number of rows in current table (ReadOnly) | Subheading | Text displayed once at the beginning the Table, below heading | SubheadingFont | FontType for Table Subheading (default is null to use working ParagraphStyle) |
Table MethodsAddCSV | Flow a CSV array into table | AddColumnCells | Append cells to current column | AddColumns | Flow an array of cells into table as columns, starting from current cell. | AddRowCells | Append cells to current row. | AddRows | Flow an array of cells into table as rows, starting from current cell. | NextCell | Move cell pointer to arbitrary cell | NextColumn | Move cell pointer to the next column on the right | NextEmptyColumn | Move cell pointer after next non-empty column. | NextEmptyRow | Move cell pointer after next non-empty row. | NextRow | Move cell pointer down one row. | SetCellAlignment | Set horizontal alignment of table cells (defaults to Alignment.Left) | SetCellBox | Set cell boxes to cycle through from current cell on | SetCellClip | Set column right-edge clipping as boolean flags (defaults to true) | SetCellFillChar | Set character used to fill cell text up to the column right edge (defaults to whitespace). | SetCellFont | Set cell fonts to cycle through from current cell on | SetCellMargins | Set margins above/below/left/right cell content within cell boundary for all table cells (default is 3) | SetCellText | Set a specific cell text, extending table size if necessary. | SetCellVerticalAlignment | Set the vertical alignment of table cells (default is Top) | SetCellWrap | Set text wrapping within columns as boolean flags (true by default) | SetColumnAfter | Set column right margins to cycle through from current column on (default is 3) | SetColumnBefore | Set column left margins to cycle through from current column on (default is 3) | SetColumnGrid | Set vertical grid decoration (Defaults to 0.1 black solid) | SetColumnMaxWidths | Set maximum column widths to cycle through from current column on (defaults to -1 for no limit) | SetColumnMerge | Set vertical cell merging flags to cycle through from current column on (defaults to false) | SetColumnMinWidths | Set minimum column widths to cycle through from current column on (defaults to 6 – twice the default cell gutter) | SetColumnRetain | Add indices of table columns that should be repeated on all subpages | SetColumnWidths | Set column widths to cycle through from current column on | SetOffset | Set column and row offset of table boxing and grid. | SetRowAfter | Set row bottom margins to cycle through from current row on (default is 3) | SetRowBefore | Set row top margins to cycle through from current row on (default is 3) | SetRowGrid | Set horizontal grid decoration (defaults to 0.1 black solid) | SetRowHeights | Set fixed row heights to cycle through from current row on | SetRowMaxHeights | Set maximum row heights to cycle through from current row on (defaults to -1 for no limit) | SetRowMerge | Set horizontal cell merging flags to cycle through from current row on (defaults to false) | SetRowMinHeights | Set minimum row heights to cycle through from current row on (defaults to 6 – twice the default cell gutter) | SetRowRetain | Add indices of table rows that should be repeated on all subpages | SetTitles | Set the number of leading columns and rows that are titles. | |
See also
Table Tutorials
RequirementsNamespace: Causeway Assembly: SharpPlot (in sharpplot.dll)
Send comments on this topic © Dyalog Ltd 2021
|